Canvas를 활용한 작업을 할 때는 반드시 DOM에 canvas가 렌더링 된 이후이어야 한다.
javascript를 사용중이라면 onLoad 이후, react를 사용중이라면 useEffect에서 canvas 작업을 진행해야 한다.
1. Draw Lines
export const First = () => {
// definition
const canvasRef = useRef<HTMLCanvasElement>(null);
useEffect(() => {
const context = canvasRef.current?.getContext("2d");
if (!context) return;
context.clearRect(0, 0, 800, 600);
context.fillStyle = "blue";
context.fillRect(10, 40, 30, 70);
context.fillStyle = "yellow";
context.fillRect(50, 40, 30, 70);
// Draw line steps
context.beginPath(); // reset the context state
context.strokeStyle = "red"; // set the color of the line
context.lineWidth = 10; // set the width of the line
context.moveTo(30, 70); // moveTo(x, y) - start a new path
context.lineTo(130, 70); // line(x, y) - end a new path
context.stroke(); // draw the line
});
return (
<Wrapper>
<MyFirstCanvas ref={canvasRef}>
This is fallback message for old browsers
</MyFirstCanvas>
</Wrapper>
);
};

2. Drawing Complex Lines
export const Second = () => {
// definition
const canvasRef = useRef<HTMLCanvasElement>(null);
useEffect(() => {
const context = canvasRef.current?.getContext("2d");
if (!context) return;
context.clearRect(0, 0, 800, 600);
context.beginPath();
context.strokeStyle = "red";
context.moveTo(30, 30);
context.lineTo(80, 80);
context.lineTo(130, 30);
context.lineTo(180, 80);
context.lineTo(230, 30);
context.stroke();
});
return (
<Wrapper>
<MyFirstCanvas ref={canvasRef}>
This is fallback message for old browsers
</MyFirstCanvas>
</Wrapper>
);
};
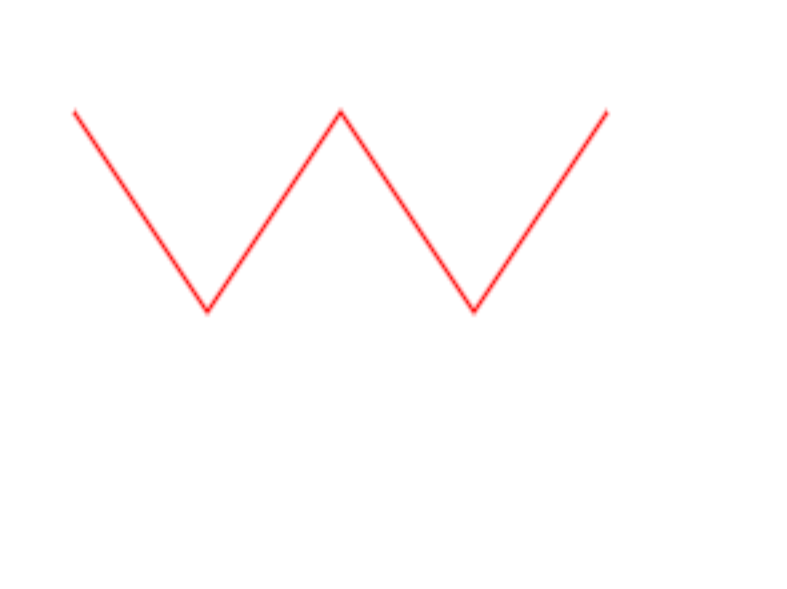
Canvas를 활용한 작업을 할 때는 반드시 DOM에 canvas가 렌더링 된 이후이어야 한다.
javascript를 사용중이라면 onLoad 이후, react를 사용중이라면 useEffect에서 canvas 작업을 진행해야 한다.
1. Draw Lines
export const First = () => {
// definition
const canvasRef = useRef<HTMLCanvasElement>(null);
useEffect(() => {
const context = canvasRef.current?.getContext("2d");
if (!context) return;
context.clearRect(0, 0, 800, 600);
context.fillStyle = "blue";
context.fillRect(10, 40, 30, 70);
context.fillStyle = "yellow";
context.fillRect(50, 40, 30, 70);
// Draw line steps
context.beginPath(); // reset the context state
context.strokeStyle = "red"; // set the color of the line
context.lineWidth = 10; // set the width of the line
context.moveTo(30, 70); // moveTo(x, y) - start a new path
context.lineTo(130, 70); // line(x, y) - end a new path
context.stroke(); // draw the line
});
return (
<Wrapper>
<MyFirstCanvas ref={canvasRef}>
This is fallback message for old browsers
</MyFirstCanvas>
</Wrapper>
);
};

2. Drawing Complex Lines
export const Second = () => {
// definition
const canvasRef = useRef<HTMLCanvasElement>(null);
useEffect(() => {
const context = canvasRef.current?.getContext("2d");
if (!context) return;
context.clearRect(0, 0, 800, 600);
context.beginPath();
context.strokeStyle = "red";
context.moveTo(30, 30);
context.lineTo(80, 80);
context.lineTo(130, 30);
context.lineTo(180, 80);
context.lineTo(230, 30);
context.stroke();
});
return (
<Wrapper>
<MyFirstCanvas ref={canvasRef}>
This is fallback message for old browsers
</MyFirstCanvas>
</Wrapper>
);
};
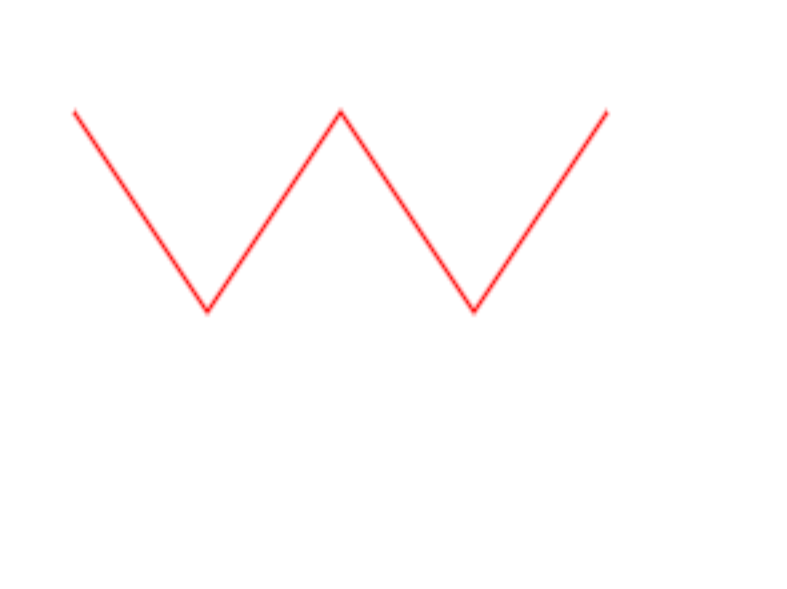